Building Modern Web Applications with .NET Minimal APIs, Blazor, and EF Core
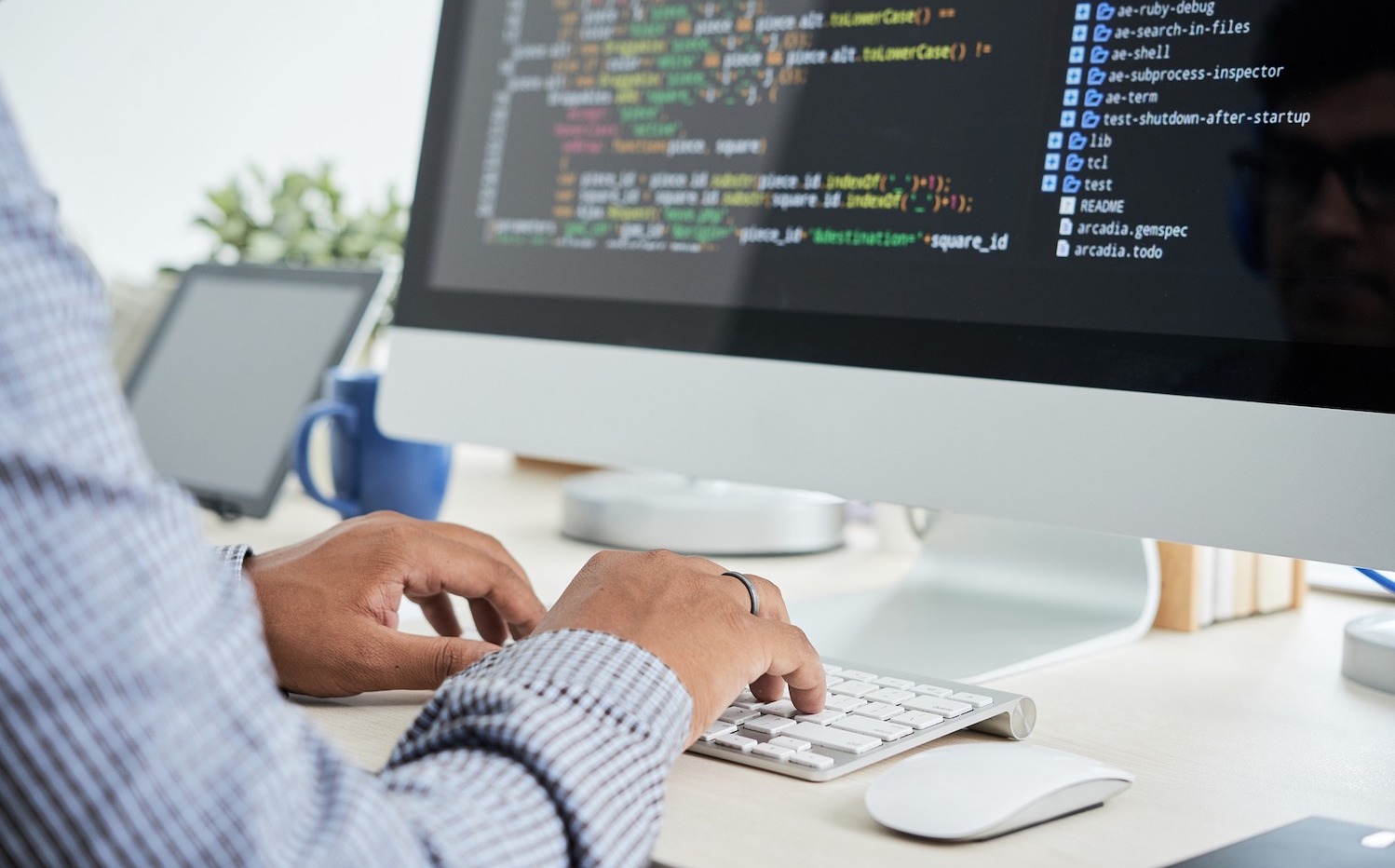
Introduction
Many enterprises struggle with legacy applications that are outdated, slow, and difficult to maintain. These applications often suffer from performance bottlenecks, security vulnerabilities, and scalability limitations due to their reliance on monolithic architectures and older technologies. As businesses evolve, they need modern, cloud-native solutions that provide better efficiency and user experience.
At QloudX, we help organizations modernize their legacy applications using cutting-edge .NET Minimal APIs, Blazor WebAssembly, and EF Core. By adopting AWS cloud services, we provide solutions that enhance scalability, performance, and security while reducing maintenance costs. This blog explores how these technologies address common challenges and how we implement them effectively.
Customer Problem
Many enterprises today face significant challenges with legacy applications, which are outdated, hard to maintain, and lack modern features. These issues impact scalability, performance, and security. Some common problems include:
- Browser Compatibility Issues – Older applications rely on outdated browsers, limiting accessibility and security.
- Scalability Constraints – Monolithic architectures make it difficult to scale applications efficiently.
- Slow Feature Implementation – Legacy systems require extensive manual intervention for updates.
- Security Risks – Older authentication mechanisms are vulnerable to modern threats.
QloudX Solution
At QloudX, we specialize in modernizing legacy applications by leveraging cutting-edge .NET technologies. Our structured approach ensures minimal disruption while improving efficiency, security, and scalability. Our solution includes:
1. Building Minimal APIs for Backend Services
Minimal APIs in .NET provide a lightweight approach to building RESTful services.
var builder = WebApplication.CreateBuilder(args); builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
app.MapGet(“/api/products”, async (AppDbContext db) => await db.Products.ToListAsync());
app.Run();
- Lightweight and Fast – Requires fewer dependencies, making API calls faster.
- Easier Routing – Cleaner syntax for defining endpoints without controllers.
- Flexible Middleware Support – Can integrate seamlessly with authentication, logging, and exception handling mechanisms.
2. Efficient Data Handling with EF Core
Optimizing database interactions is key to improving application performance. Using asynchronous queries and compiled models in EF Core significantly boosts query performance.
builder.Services.AddDbContext<AppDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString(“DefaultConnection”)));
- Async Queries – Prevents blocking the main thread, improving application responsiveness.
- Compiled Queries – Reduces execution time for frequently used queries.
- Migrations – Ensures smooth database schema evolution over time.
3. Frontend Development with Blazor WebAssembly
Blazor WebAssembly provides a C#-based frontend framework, eliminating the need for JavaScript-heavy SPA frameworks like Angular or React.
@code {
private string message = “Hello, Blazor!”;
}
<h3>@message</h3>
<button @onclick=”() => message = ‘Button Clicked!'”>Click Me</button>
- Component-Based Architecture – Reusable components simplify UI development.
- Full-Stack C# Development – Allows sharing models and logic between frontend and backend.
- WebAssembly Execution – Runs client-side for faster interactions.
Architecture Overview
To ensure scalability and maintainability, we structured our application as follows:
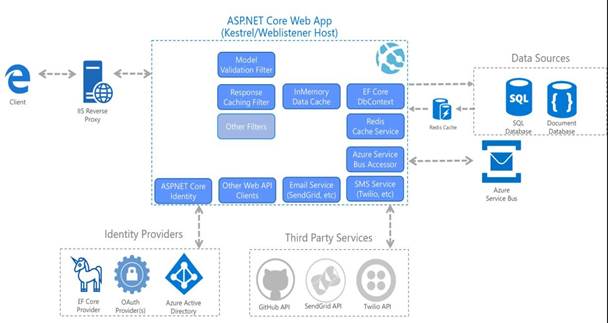
This architecture ensures high availability, modular development, and scalable cloud deployment using AWS services such as API Gateway, Lambda, and RDS.
Business Value Delivered
By adopting Minimal APIs, Blazor WebAssembly, and EF Core, we successfully modernized applications, delivering:
- 50% Faster API Response Times – Optimized data access and processing.
- Scalable s Cloud-Ready Applications – Built with AWS cloud-native services.
- Reduced Maintenance Overhead – Automated testing and CI/CD reduced manual interventions.
- Enhanced User Experience – Intuitive UI with fast rendering and cross-platform compatibility.
Best Practices for Developers
- Optimize API Performance – Use caching mechanisms (Redis), minimize payload size, and implement rate limiting.
- Use Dependency Injection – Keeps services loosely coupled and easier to test.
- State Management in Blazor – Leverage local storage, session storage, or Flux-like patterns.
- Security Best Practices – Implement OAuth2, JWT authentication, and secure API endpoints with proper authorization policies.
Lessons Learned
- Minimal APIs make backend development faster – Reduced boilerplate makes services cleaner and more maintainable.
- EF Core’s async capabilities are a must for performance – Improves database efficiency significantly.
- Blazor WebAssembly bridges the frontend-backend gap – Full-stack C# development eliminates JavaScript dependencies.
- CI/CD integration is crucial for production readiness – Automating deployments ensures reliability and efficiency.
Conclusion
Modernizing web applications with Minimal APIs, Blazor WebAssembly, and EF Core significantly enhances performance, scalability, and maintainability. By leveraging AWS cloud services, businesses can ensure long-term sustainability, security, and improved user experience.
If your organization is struggling with outdated applications, let’s discuss how QloudX can help you modernize and future-proof your software.
About the Author
Sharique Anwar is a .NET developer with over 4 years of experience in software development. A graduate from IIT BHU, he specializes in .NET development, Blazor WebAssembly, Minimal APIs, and EF Core. Since joining QloudX in January 2022, Sharique has contributed to multiple modernization projects focusing on scalable cloud-native solutions. He holds AWS Foundation and Associate Developer certifications, demonstrating his expertise in cloud technologies and high-performance application development